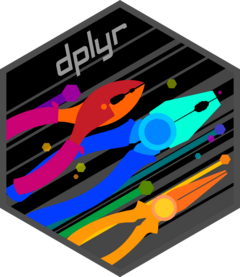
Package index
-
arrange()
- Order rows using column values
-
distinct()
- Keep distinct/unique rows
-
filter()
- Keep rows that match a condition
-
slice()
slice_head()
slice_tail()
slice_min()
slice_max()
slice_sample()
- Subset rows using their positions
-
glimpse
- Get a glimpse of your data
-
mutate()
- Create, modify, and delete columns
-
pull()
- Extract a single column
-
relocate()
- Change column order
-
rename()
rename_with()
- Rename columns
-
select()
- Keep or drop columns using their names and types
-
count()
tally()
add_count()
add_tally()
- Count the observations in each group
-
group_by()
ungroup()
- Group by one or more variables
-
dplyr_by
- Per-operation grouping with
.by
/by
-
rowwise()
- Group input by rows
-
summarise()
summarize()
- Summarise each group down to one row
-
reframe()
experimental - Transform each group to an arbitrary number of rows
-
n()
cur_group()
cur_group_id()
cur_group_rows()
cur_column()
- Information about the "current" group or variable
-
bind_cols()
- Bind multiple data frames by column
-
bind_rows()
- Bind multiple data frames by row
-
intersect()
union()
union_all()
setdiff()
setequal()
symdiff()
- Set operations
-
inner_join()
left_join()
right_join()
full_join()
- Mutating joins
-
nest_join()
- Nest join
-
semi_join()
anti_join()
- Filtering joins
-
cross_join()
- Cross join
-
join_by()
- Join specifications
-
rows_insert()
rows_append()
rows_update()
rows_patch()
rows_upsert()
rows_delete()
- Manipulate individual rows
Multiple columns
Pair these functions with mutate()
, summarise()
, filter()
, and group_by()
to operate on multiple columns simultaneously.
-
c_across()
- Combine values from multiple columns
-
pick()
- Select a subset of columns
Vector functions
Unlike other dplyr functions, these functions work on individual vectors, not data frames.
-
between()
- Detect where values fall in a specified range
-
case_match()
- A general vectorised
switch()
-
case_when()
- A general vectorised if-else
-
coalesce()
- Find the first non-missing element
-
consecutive_id()
- Generate a unique identifier for consecutive combinations
-
desc()
- Descending order
-
if_else()
- Vectorised if-else
-
n_distinct()
- Count unique combinations
-
na_if()
- Convert values to
NA
-
near()
- Compare two numeric vectors
-
ntile()
- Bucket a numeric vector into
n
groups
-
order_by()
- A helper function for ordering window function output
-
percent_rank()
cume_dist()
- Proportional ranking functions
-
recode()
recode_factor()
superseded - Recode values
-
row_number()
min_rank()
dense_rank()
- Integer ranking functions
-
band_members
band_instruments
band_instruments2
- Band membership
-
starwars
- Starwars characters
-
storms
- Storm tracks data
Grouping helpers
This (mostly) experimental family of functions are used to manipulate groups in various ways.
-
group_cols()
- Select grouping variables
-
group_map()
group_modify()
group_walk()
experimental - Apply a function to each group
-
group_trim()
experimental - Trim grouping structure
Superseded
Superseded functions have been replaced by new approaches that we believe to be superior, but we don’t want to force you to change until you’re ready, so the existing functions will stay around for several years.
-
sample_n()
sample_frac()
superseded - Sample n rows from a table
-
top_n()
top_frac()
superseded - Select top (or bottom) n rows (by value)
-
scoped
superseded - Operate on a selection of variables
-
all_vars()
any_vars()
superseded - Apply predicate to all variables
-
vars()
superseded - Select variables
-
with_groups()
superseded - Perform an operation with temporary groups
-
auto_copy()
- Copy tables to same source, if necessary
-
compute()
collect()
collapse()
- Force computation of a database query
-
copy_to()
- Copy a local data frame to a remote src
-
ident()
- Flag a character vector as SQL identifiers
-
explain()
show_query()
- Explain details of a tbl
-
sql()
- SQL escaping.